Overview
HealthBook is for clinic receptionist who prefer to use a desktop app for managing doctors and patients information and appointments. It is optimized for receptionist who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
Summary of contributions
-
Major enhancement: Added the command to register both doctors and patients
-
What it does: This allows the user to register new doctors and patients into the HealthBook.
-
Justification: This feature enables the user to add new doctors and patients into the HealthBook so that further commands can be called on them.
-
Highlights: This enhancement is required for all other commands of the HealthBook to work. It required an in-depth considerations of the data structure to store the information of patients and doctors. The implementation required a good understanding of what was already implemented in the previous versions and what is required for further implementations of subsequent commands and usage.
-
-
Major enhancement: Added the command to complete appointments
-
What it does: This allows the user to complete upcoming appointments of patients.
-
Justification: This feature enables the user to keep track of the upcoming and past appointments in the HealthBook, and updates the doctors' calendars at the same time.
-
Highlights: This enhancement is the primary purpose of the HealthBook. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required integration with Google calendar commands.
-
-
Major enhancement: Integrated Google Calendar into HealthBook
-
What it does: This allows information of appointments to be presented to doctors in their Google calendar when appointments are added, completed or deleted. They will be able to review the patient they are seeing as well as time of the appointment easily.
-
Justification: This feature allows doctors to receive information about their upcoming appointments in a decentralized manner. Receptionists can use this integration to pass information to doctors that may not be in the clinic at that point in time.
-
Highlights: This enhancement required a good understanding of both integration of Google’s API and other commands of the HealthBook. It required an extensive understanding of integration in Java and the Google’s API that is available. In addition, this implementation required a good analysis of how the Google Calendar can compliment the features in the HealthBook.
-
Credits: Google’s API https://developers.google.com/resources/api-libraries/documentation/calendar/v3/java/latest/
-
-
Other contributions:
-
Project management:
-
Setting up of Jar files for Release.
-
Assigning issues and datelines after v1.3.
-
-
Implementation of new features:
-
Added
register-patient
andregister-doctor
commands (Pull requests #15) -
Added
complete-appointment
command (Pull requests #62) -
Integrated Google Calendar to
add-appointment
,delete-appointment
andcomplete-appointment
commands (Pull requests #54, #27) -
Modified storage to accommodate patients, doctors, appointments and prescriptions. (Pull requests #32)
-
Wrote test cases for changes in models (Pull requests #76)
-
Wrote test cases for changes in storage (Pull requests #59)
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Registering a patient: register-patient
Registers a patient to the HealthBook. Patient is automatically tagged as a "Patient".
Format: register-patient n/NAME p/PHONE_NUMBER e/EMAIL a/ADDRESS
Examples:
-
register-patient n/John Doe p/98765432 e/johnd@example.com a/John street, block 123, #01-01
After registering the patient, all commands that requires the patient’s name are non-case sensitive for convenience. |
Registering a doctor: register-doctor
Registers a doctor to the HealthBook. Doctor is automatically tagged as a "Doctor".
Format: register-doctor n/NAME p/PHONE_NUMBER e/EMAIL a/ADDRESS
Login to Google Calendar is mandatory. If no login is done, the application will not be able to proceed. Please ensure login is done before proceeding back to the HealthBook. |
Examples:
-
register-doctor n/Mary Jane p/98765432 e/maryj@example.com a/John street, block 123, #01-01
After registering the doctor, all commands that requires the doctor’s name are non-case sensitive. |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Calendar component
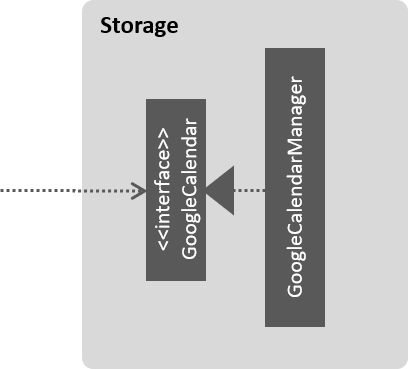
API :
GoogleCalendar.java
The Calendar
,
-
uses Google Calendar API to access the users Google calendar to read and write information to their calendar.
-
stores the OAuth of users google calendar data in tokens folder.
Model component
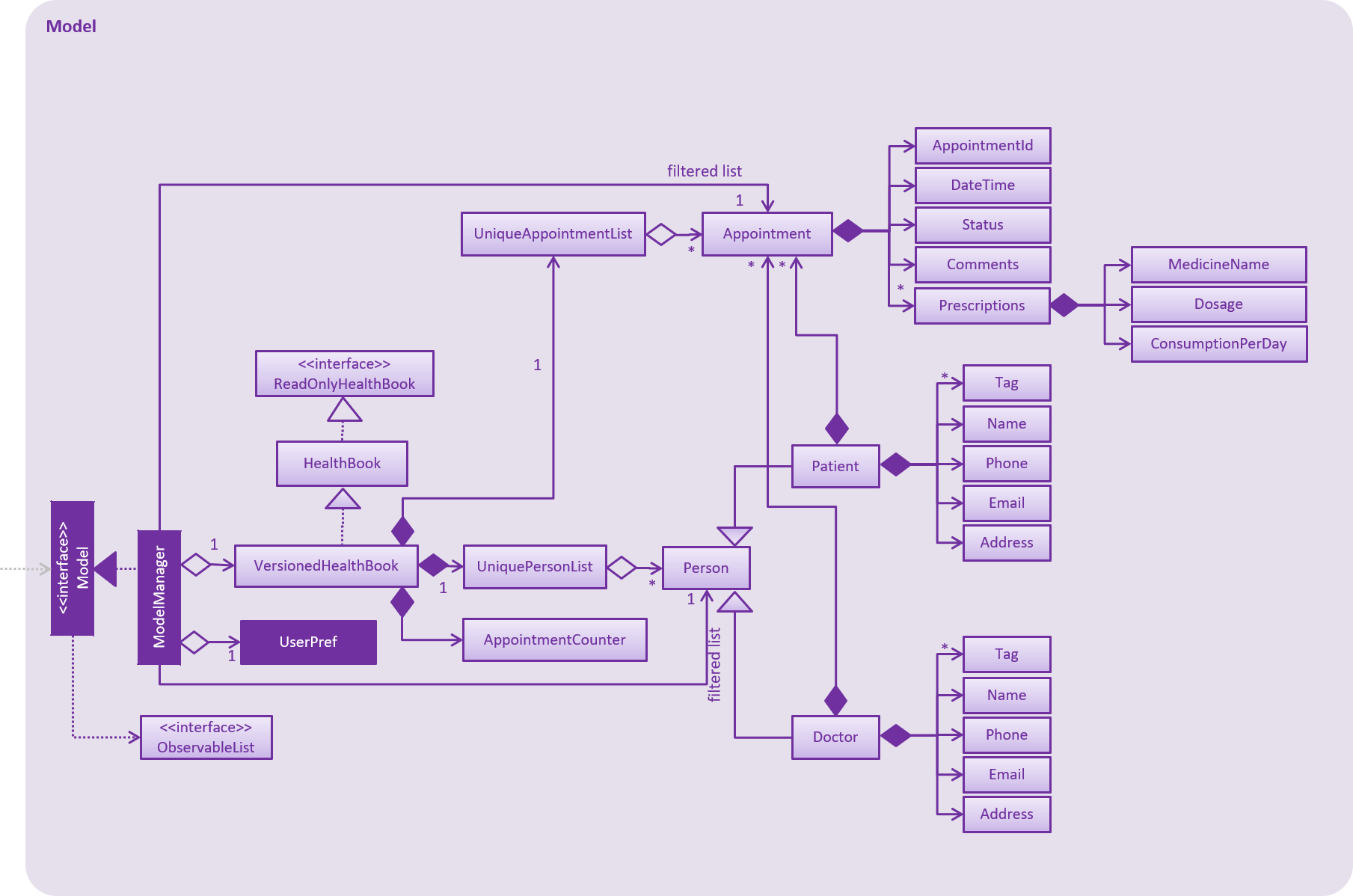
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the HealthBook data.
-
exposes an unmodifiable
ObservableList<Person>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
Storage component
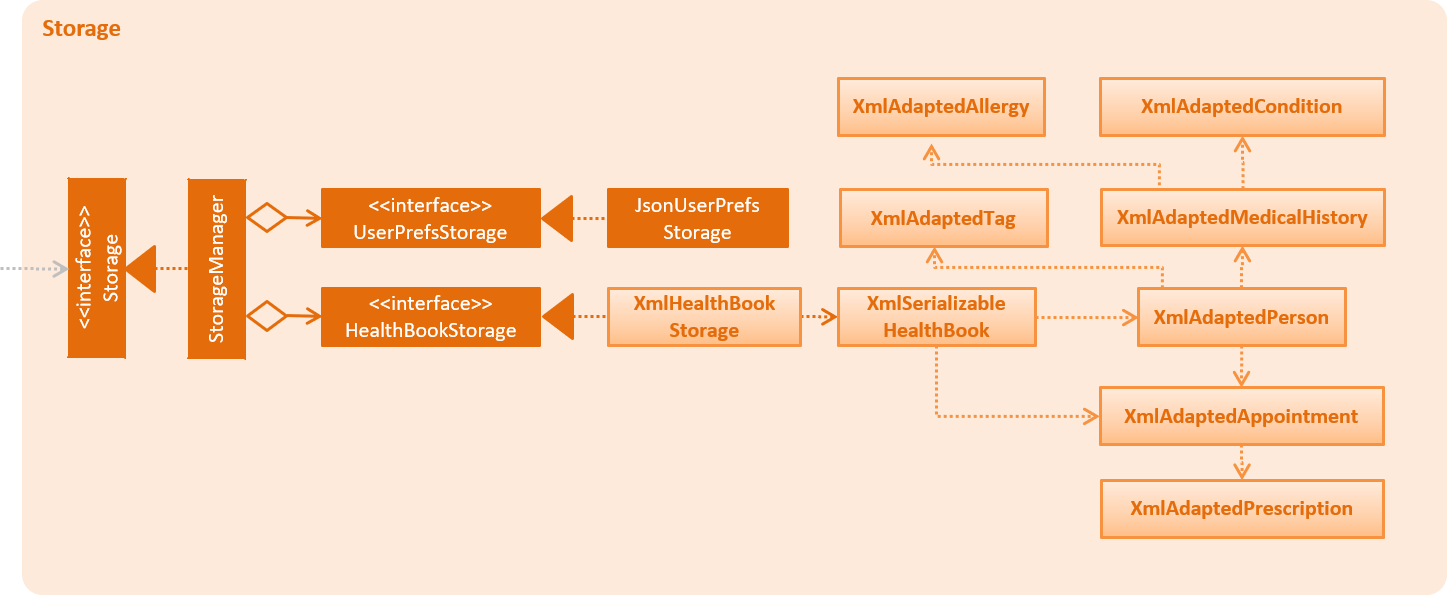
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the HealthBook data in xml format and read it back.
Register Patient Feature
Current Implementation
The register patient are facilitated by RegisterPatientCommand
and RegisterPatientCommandParser
. The Patient object is
automatically tagged as Patient
.
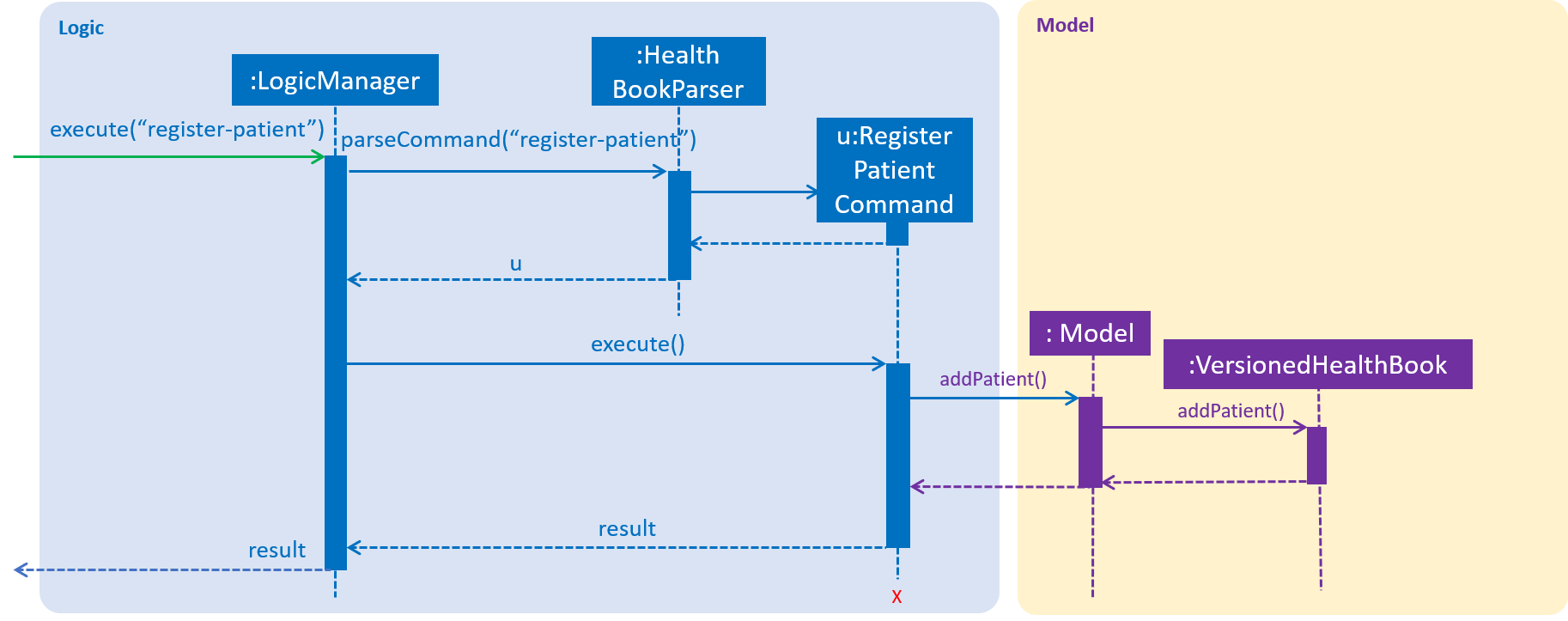
Patient model contains a List of upcoming appointment and List of past appointments. We implemented the patient model in this way to allow ease of addition of new upcoming appointment to the patient.
Step 1. The user types in register-patient
in the command box followed by the parameters n/John Doe p/91234567
e/test@test.com a/123 Clementi Road
Command Exception will be thrown if any of the information is missing or invalid. All fields are mandatory. |
Step 2. Upon hitting enter, the Patient
will be created and added to the storage of the system
Patient is saved in the storage as person. However, when we retrieve the patient object from storage, it will be created as a patient as determined by their tag. |
Design Considerations
Aspect: Data Structure of Upcoming and Past Appointments
-
Alternative 1 (Current Choice): Upcoming Appointments stored within a List and Past Appointments stored within a List. This allows the appointments to be compatible with the Observable List. Sorted list of appointments is not crucial to the implementation of the HealthBook. Hence, we chose to implement the data structure as a list instead.
-
Pros: Compatible and easily to implement with model and storage
-
Cons: List is not sorted
-
-
Alternative 2 (Alternative Choice): Upcoming Appointments stored within a PriorityQueue and Past Appointments stored within an ArrayList. This allows the appointments to be added in a non chronological order and automatically slotted in the correct position. Past appointments can also be iterated through easily when required.
-
Pros: Appointments can be added in a non chronological order.
-
Cons: Need to do parsing before passing to model and storage. Hard to manage.
-
Register Doctor Feature
Current Implementation
The register doctor are facilitated by RegisterDoctorCommand
and RegisterDoctorCommandParser
. The Doctor object is
automatically tagged as Doctor
.
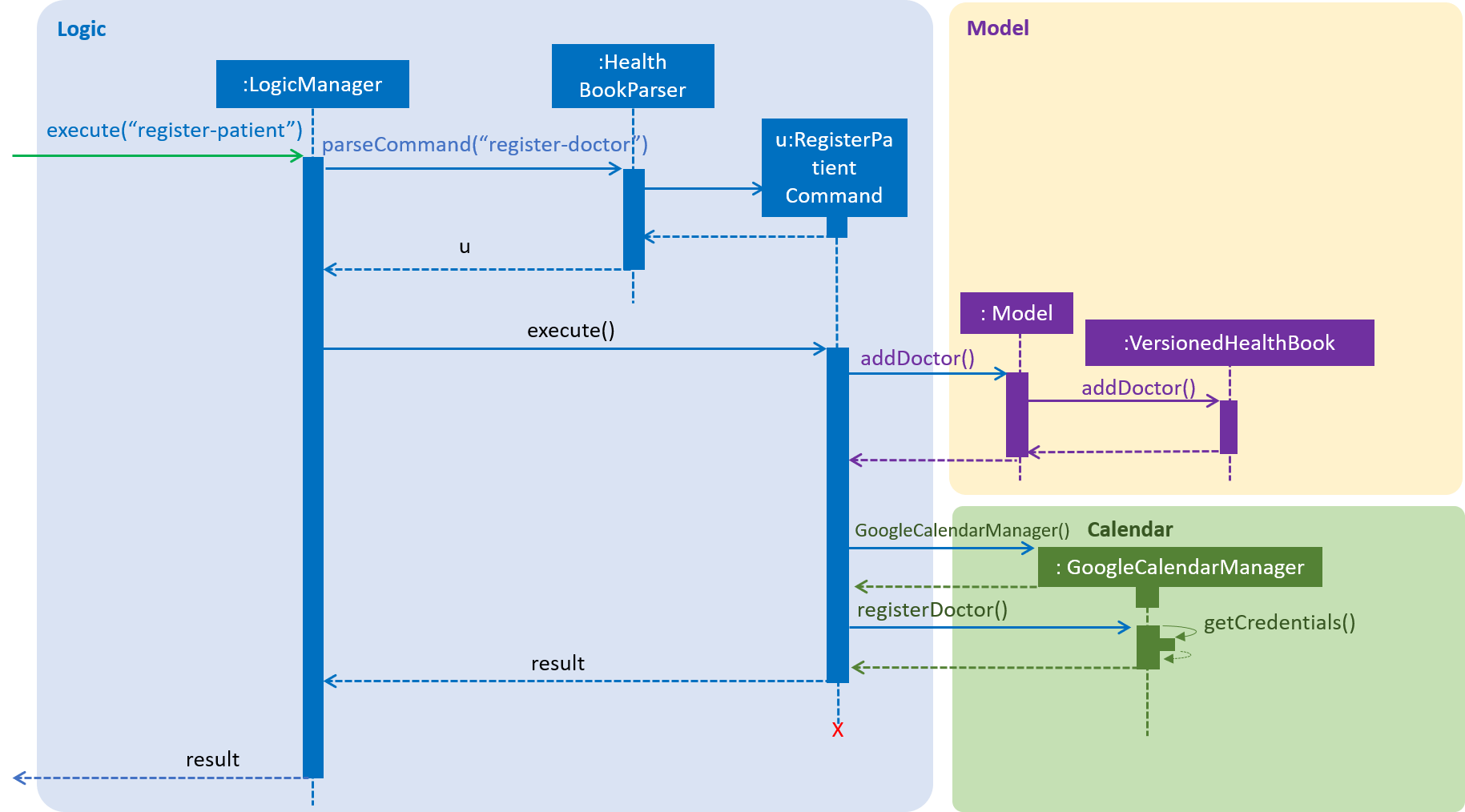
Step 1. The user types in register-doctor
in the command box followed by the parameters n/John Doe p/91234567 e/test@test.com a/123 Clementi Road
Command Exception will be thrown if any of the information is missing or invalid. All fields are mandatory. |
Step 2. Upon hitting enter, a browser will be opened for user to login to google to register their Google Calendar.
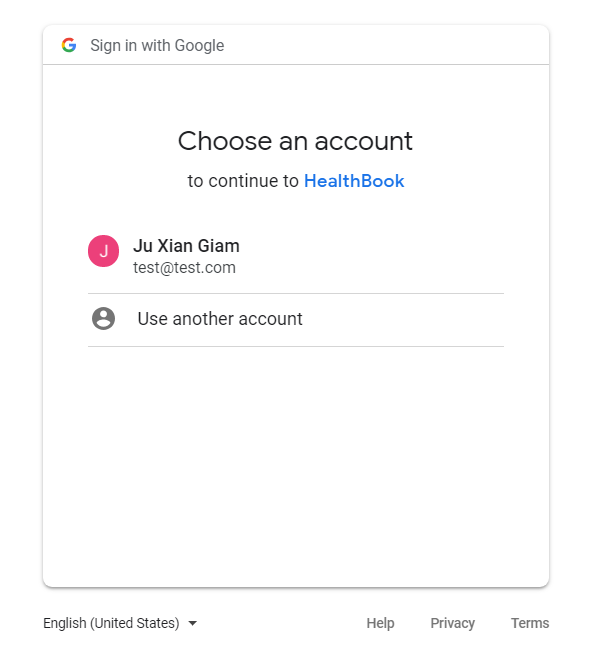
Step 3. Simultaneously, the Doctor
will be created and added to the storage of the system. In the case that the user
does not login to google, subsequent access to the HealthBook will be denied.
Login to Google Calendar is MANDATORY for registration of doctor. This is to facilitate the features that allows doctor to interact with the HealthBook. If no login is done, the HealthBook will not proceed. |
Doctor is saved in the storage as person. However, when we retrieve the doctor object from storage, it will be created as a doctor as determined by their tag. |
Design Considerations
Aspect: Presenting Google Calendar Login to Doctors
-
Alternative 1 (Current Choice): Implement Google Calendar login with a popped up browser.
-
Pros: Data transfer for the Google login to be simplified. No additional parsing will be required. In addition, the pop up of the browser will capture the users attention, decreasing the chance of user missing this login procedure.
-
Cons: Temporarily exits the application.
-
-
Alternative 2 (Alternative Choice): Implement Google Calendar login with the browser panel instead. Instead of having an additional browser, we load the login page into the Browser Panel. This will facilitate a seamless login for the login but user might miss the login compared to having a popped up browser.
-
Pros: Stays within the application.
-
Cons: User may miss the login which is mandatory, causing the application to not respond.
-
Aspect: Saving Doctor with respect to Google Login
-
Alternative 1 (Current Choice): Doctor object will not be created and application will not respond if login is unsuccessful. From the integration used for Google OAuth, it is mandatory for user to login before any process can continue. This function as our features is complimentary for our application as HealthBook will work at its best with the google calendar login.
-
Pros: No requirement to implement a new Google OAuth. Easy implementation.
-
Cons: Application may not respond if login is not done.
-
-
Alternative 2 (Alternative Choice): Allow doctor to still be created with or without the Google Login. Implement register-doctor to have Google Login as optional. However, subsequent calls of Calendar related functions will still prompt the user to login.
-
Pros: Flexible login feature.
-
Cons: Hard to implement. Need to recreate a Google OAuth system. Features of the HealthBook not utilized.
-