Overview
HealthBook is for clinic receptionist who prefer to use a desktop app for managing doctors and patients information and appointments. It is optimized for receptionist who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
Summary of contributions
-
Major enhancement: added the ability to add and delete appointments
-
What it does: This allows the user to add new appointments and remove existing appointments that are done in the HealthBook.
-
Justification: This feature enables the user to keep track of the upcoming and past appointments in the HealthBook, and updates the doctors' calendars at the same time.
-
Highlights: This enhancement is the primary purpose of the HealthBook. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required integration with Google calendar commands.
-
Credits: giamjuxian for the Google calendar integration
-
-
Minor enhancement:
-
Added autocomplete commands on tab feature
-
Added commands to view patients/doctors
-
Added commands to delete patients/doctors
-
Updated UI and events after every command call
-
-
Code contributed: Functional code
-
Other contributions:
-
Project management:
-
Managed release
v1.3
on GitHub -
Setting up AppVeyor, Travis, Coveralls and Netlify for the project
-
-
Implementation of new features:
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Deleting a patient: delete-patient
Deletes a patient from the HealthBook by name and phone.
Format: delete-patient n/NAME [p/PHONE]
Parameter p/PHONE is only needed when there are duplicate names in HealthBook, so phone number is needed to differentiate them.
|
Examples:
-
delete-patient n/Jane Doe
-
delete-patient n/John Doe p/98264293
Deleting a doctor: delete-doctor
Deletes a doctor from the HealthBook by name and phone.
Format: delete-doctor n/NAME [p/PHONE]
Parameter p/PHONE is only needed when there are duplicate names in HealthBook, so phone number is needed to differentiate them.
|
Examples:
-
delete-doctor n/Amanda Jane
-
delete-doctor n/Mary Jane p/94726274
Viewing a patient: view-patient
Views a landing page of a patient on the HealthBook. This will display all information of the patient onto the HealthBook
including their medical history, upcoming appointment and their past appointments.
Format: view-patient n/NAME [p/PHONE]
Parameter p/PHONE is only needed when there are duplicate names in HealthBook, so phone number is needed to differentiate them.
|
Examples:
-
view-patient n/John Doe
-
view-patient n/Jane Doe p/93726273
Viewing a doctor: view-doctor
Views a landing page of a doctor on the HealthBook. This will display the doctor’s upcoming appointments.
Format: view-doctor n/NAME [p/PHONE]
Parameter p/PHONE is only needed when there are duplicate names in HealthBook, so phone number is needed to differentiate them.
|
Examples:
-
view-doctor n/Amanda Jane p/93726272
-
view-doctor n/Mary Jane
Booking an appointment: add-appointment
Book a doctor’s appointment for the patient. An ID of this appointment will be generated.
Format: add-appointment np/PATIENT_NAME [pp/PATIENT_PHONE] nd/DOCTOR_NAME [pd/DOCTOR_PHONE] d/DATE_TIME
Parameter p/PHONE is only needed when there are duplicate names in HealthBook, so phone number is needed to differentiate them.
|
Examples:
-
add-appointment np/Jane Doe pp/92937272 nd/Amanda Jane pd/94827284 d/2018-12-12 13:00
-
add-appointment np/John Doe nd/Mary Jane d/2018-10-17 15:00
Deleting an appointment delete-appointment
Delete a doctor’s appointment for the patient by its ID.
Format: delete-appointment APPOINTMENT_ID
Examples:
-
delete-appointment 10001
Tab to autocomplete commands
In the command box, user can type the first few characters of the command and tab to autocomplete it.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Delete Patient and Doctor Feature
Current Implementation
The delete patient and doctor features are facilitated by DeletePatientCommand
and DeleteDoctorCommand
. Both extends
DeleteCommand
such that it is able to delete a specific subclass of Person
(i.e. Patient
or Doctor
) instead. Additionally,
instead of deletion by index, deletion by name is used in this implementation.
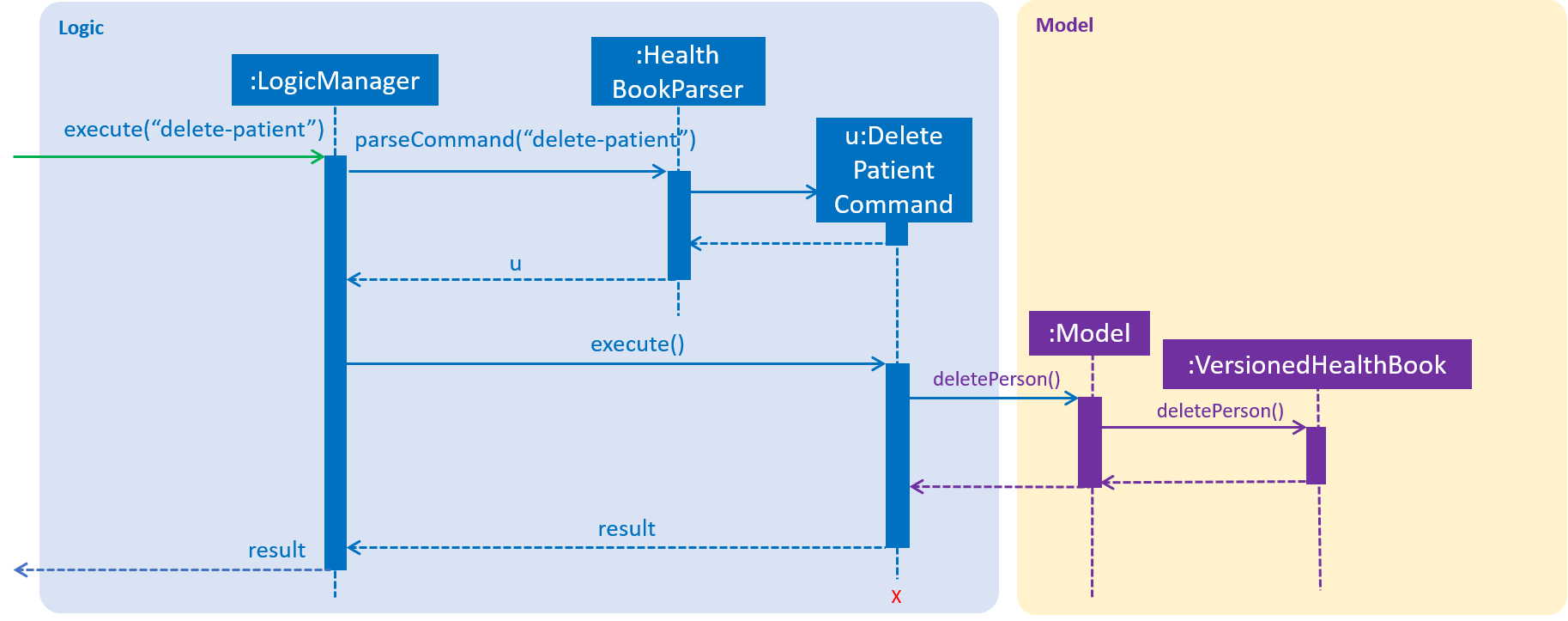
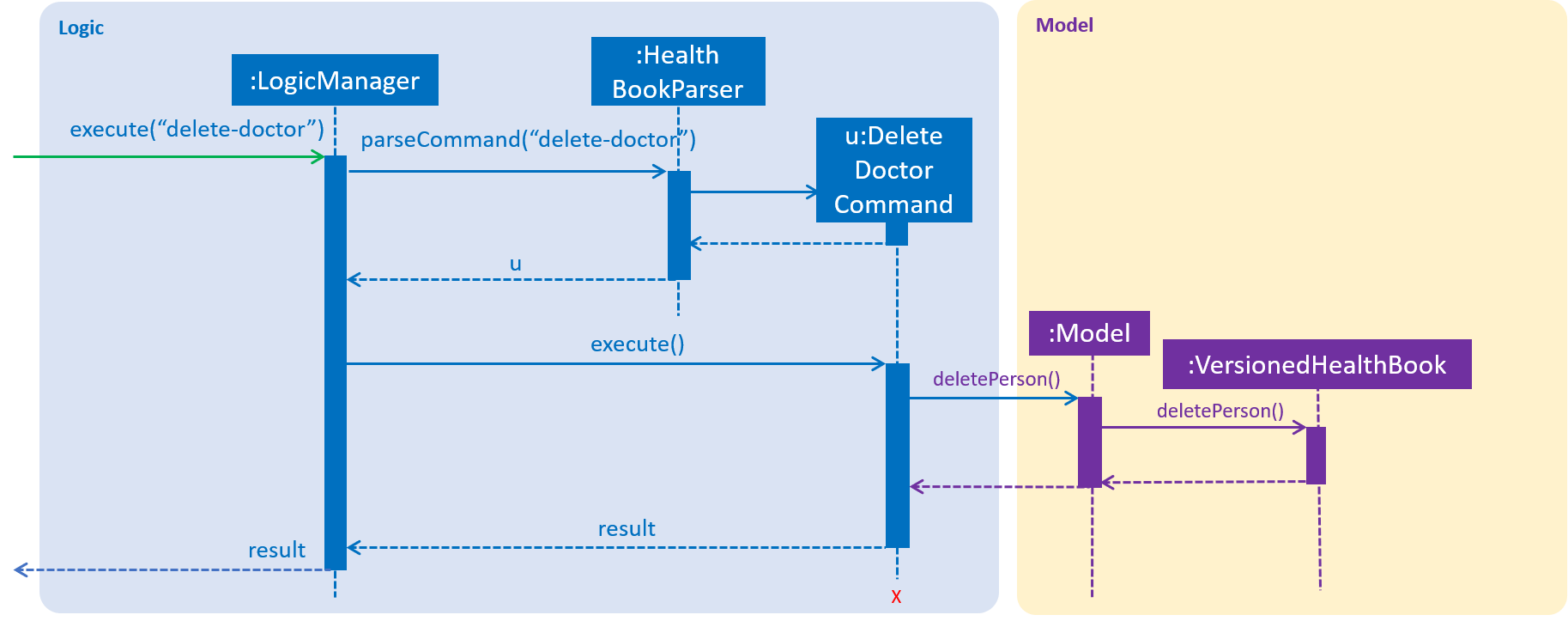
Step 1. The user types in delete-patient
or delete-doctor
in the command box followed by the parameters n/John Doe p/12345678
Step 2. Upon hitting enter, the Patient
or Doctor
will be removed from the system.
Design Considerations
Aspect: Algorithm to find Patient
or Doctor
to delete
-
Alternative 1 (Current choice): Loop through
model.getFilteredPersonList()
to findPatient
orDoctor
to delete-
Pros: Easy to implement
-
Cons: If size of list grows unusually large (unusual because of our target audience), it may take awhile to retrieve the
Patient
orDoctor
-
-
Alternative 2: Use a Map that maps name to
Patient
orDoctor
-
Pros: Fast retrieval
-
Cons: Will have to change a lot of lines of code
-
View Patient and Doctor Feature
Current Implementation
The view patient and doctor features are facilitated by ViewPatientCommand
and ViewDoctorCommand
. This feature shows information
of the person corresponding to the name specified.
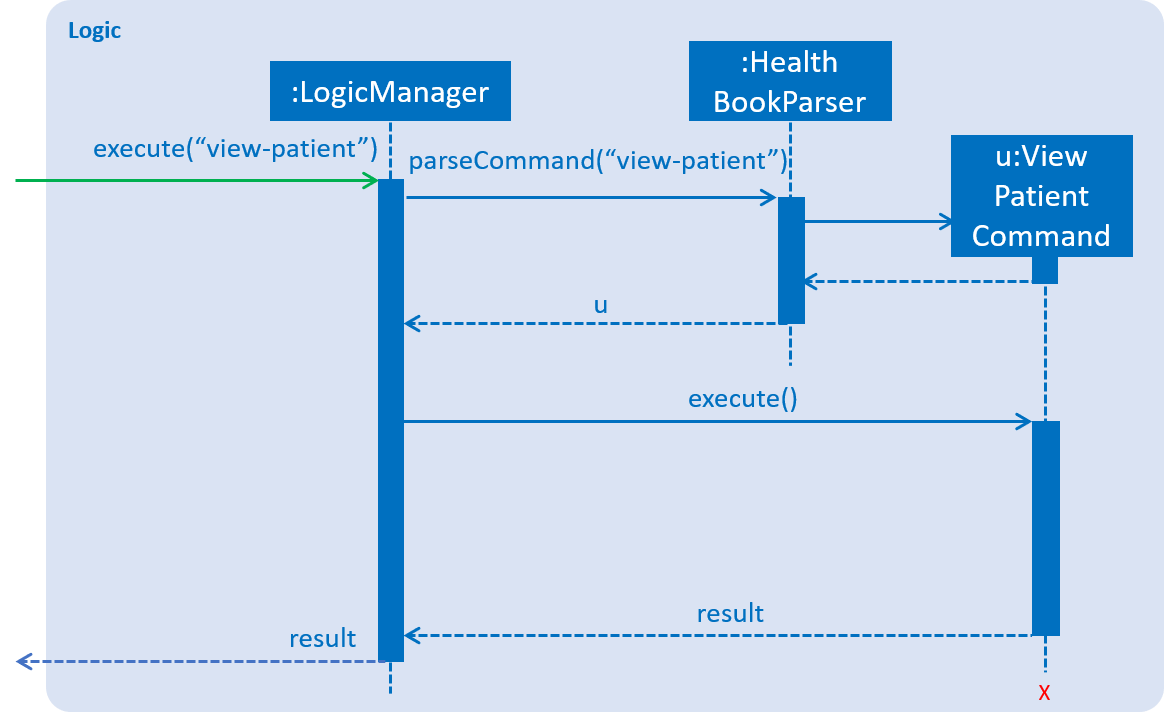
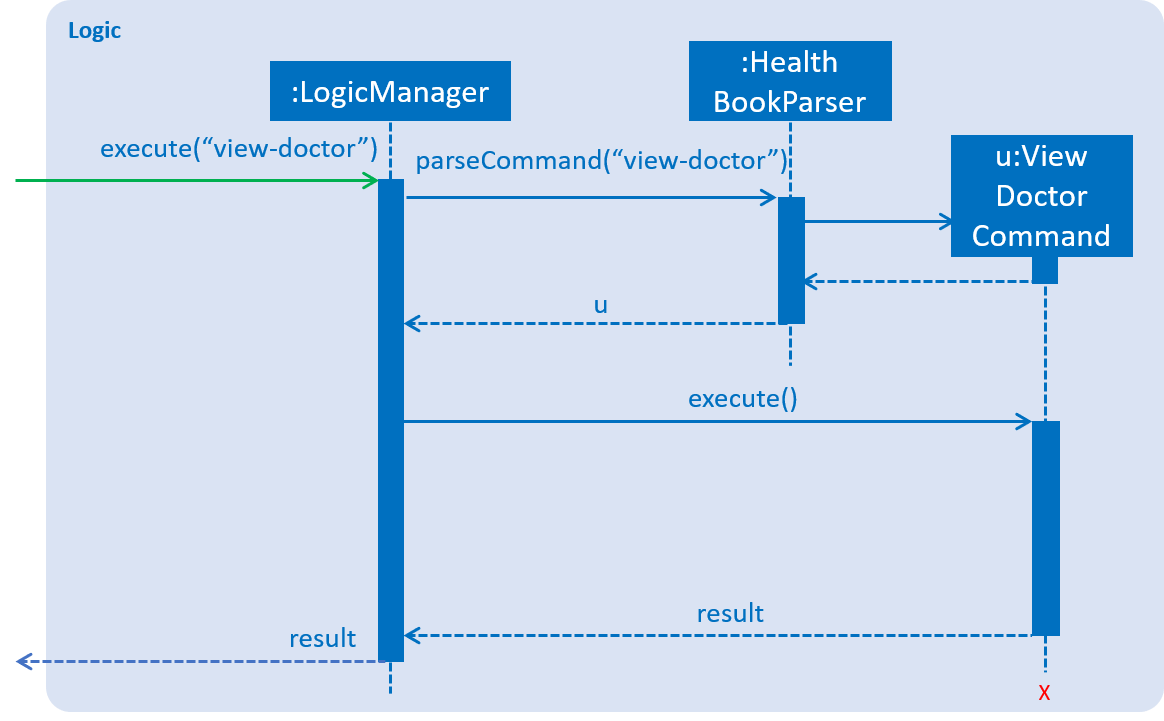
Step 1. The user types in view-patient
or view-doctor
in the command box followed by the parameters n/John Doe
Step 2. Upon hitting enter, information of Patient
or Doctor
will be reflected on the main information panel
Add Appointment Feature
Current Implementation
The add appointment features are facilitated by AddAppointmentCommand
. This feature allows user to add appointments in the HealthBook
which will be added to the respective Patient
and Doctor
of the Appointment
as well.
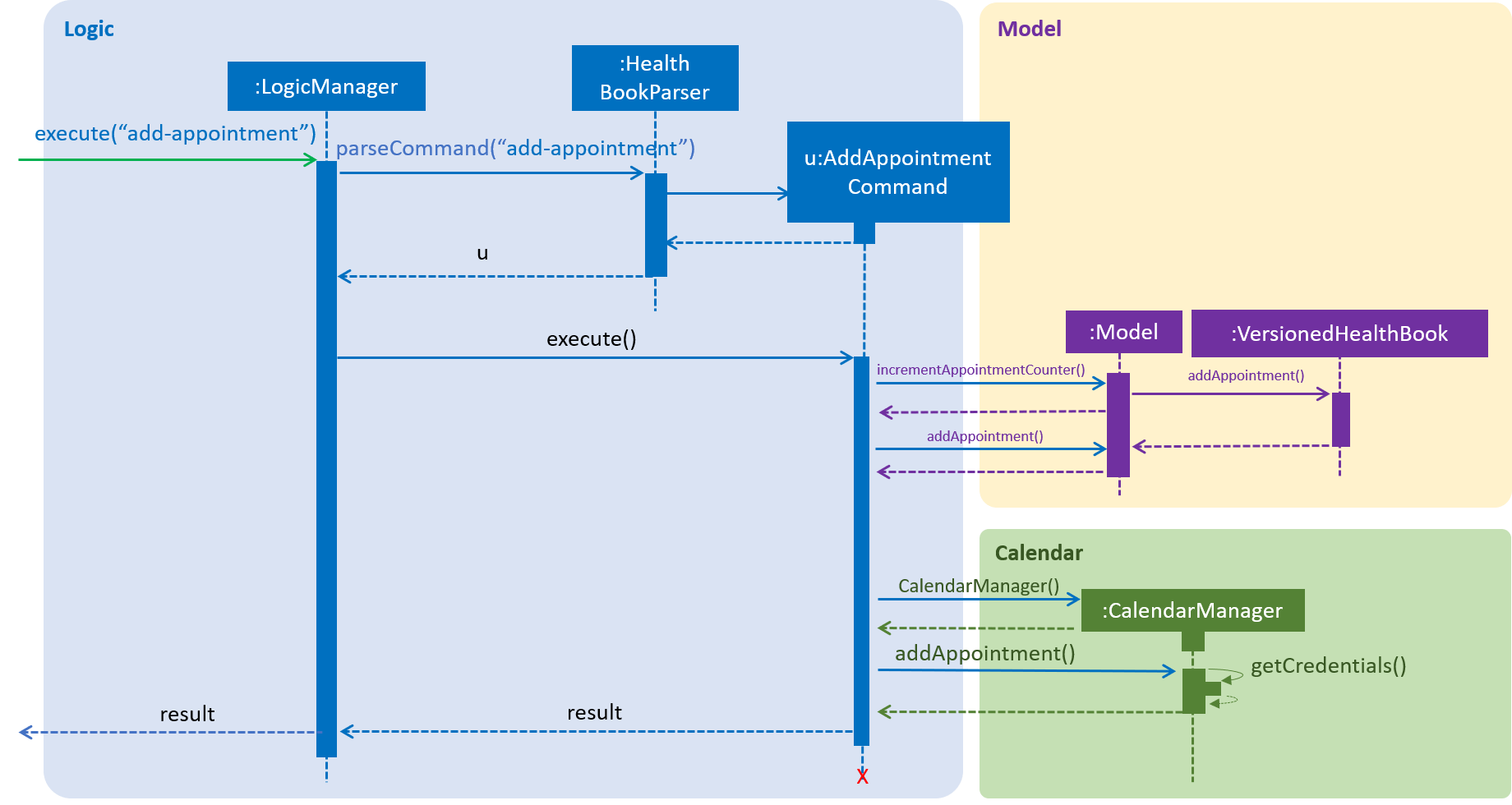
Step 1. The user types in add-appointment
in the command box followed by the parameters np/John Doe nd/Mary Jane d/2018-10-17 15:00
Step 2. Upon hitting enter, the Appointment
will be added to the HealthBook, the Patient
, and the Doctor
and his/her Google calendar
Delete Appointment Feature
Current Implementation
The delete appointment features are facilitated by DeleteAppointmentCommand
. This feature allows user to delete appointments in the HealthBook
which will be deleted from the respective Patient
and Doctor
of the Appointment
as well.
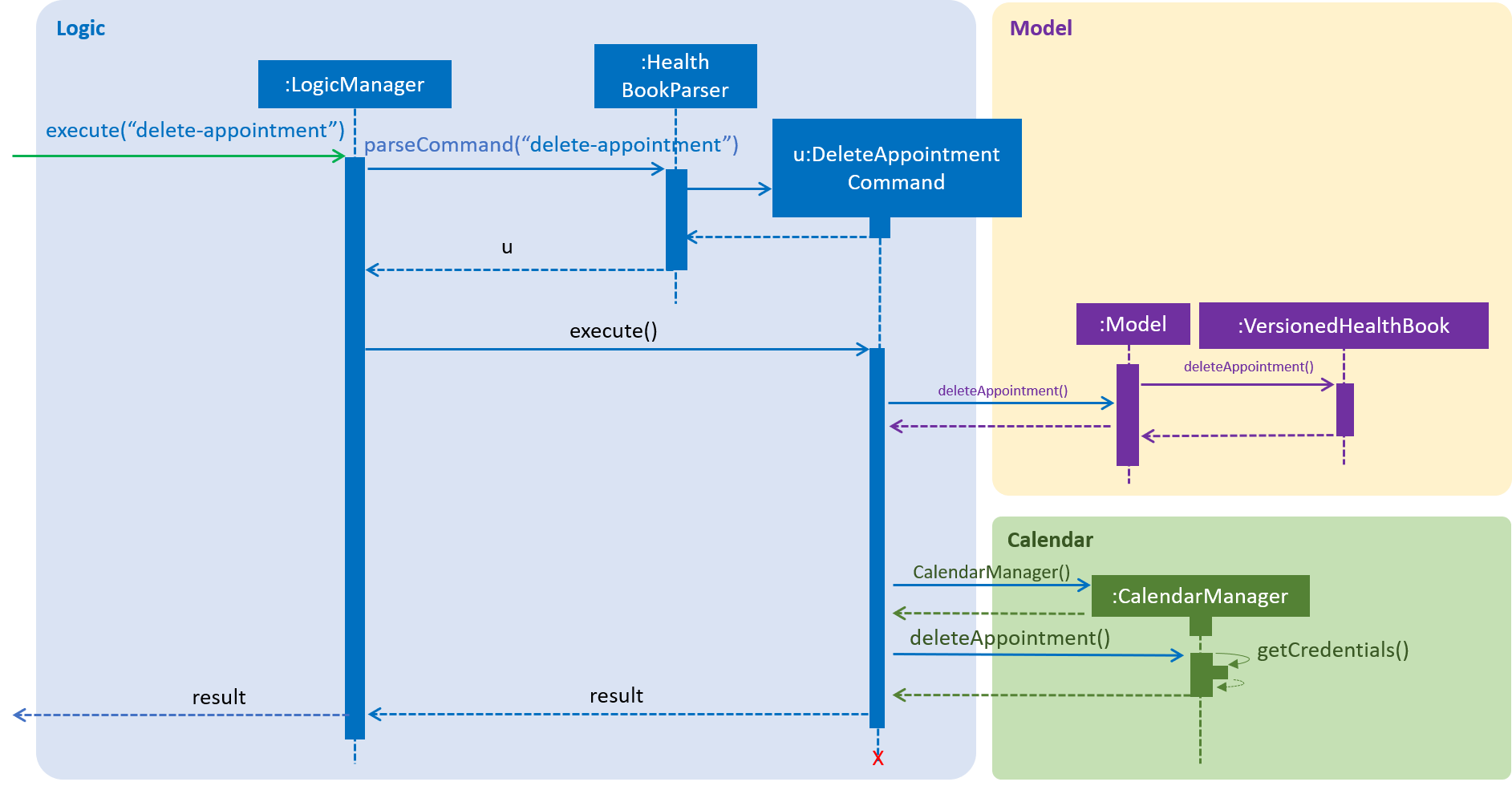
Step 1. The user types in delete-appointment
in the command box followed by the appointment id 10000
Step 2. Upon hitting enter, the Appointment
will be removed from the HealthBook, the Patient
, and the Doctor
and his/her Google calendar
Appendix A: Glossary
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Receptionist
-
Target audience
- Patient
-
Indirect target audience, patient whom visits the clinic
- Doctor
-
Indirect target audience, doctor whom works at the clinic
- Appointment
-
A date and time fixed for a patient to consult a doctor
- Prescription
-
The medicine prescribed for the appointment
- Medical History
-
Allergies and/or conditions that a patient may have
Appendix B: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Deleting a patient
-
Deleting a patient
-
Prerequisites: List all persons using the
list
command iffilter-patient
orfilter-doctor
has been called before. -
Test case:
delete-patient n/John Doe
Expected: Patient named John Doe deleted from the HealthBook, along with any appointments tied to him. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete-patient n/jlfsajfl
Expected: No patient is deleted if no patient with such name exists. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete-patient
Expected: Similar to previous.
-
Viewing a patient
-
Viewing a patient
-
Prerequisites: List all persons using the
list
command iffilter-patient
orfilter-doctor
has been called before. -
Test case:
view-patient n/John Doe
Expected: Information panel reflects information of John Doe from the HealthBook. Name of patient currently viewing is shown in the status message. Timestamp in the status bar is updated. -
Test case:
view-patient n/jlfsajfl
Expected: No patient is viewed if no patient with such name exists. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
view-patient
Expected: Similar to previous.
-